How to Delete a Whole Taxonomy from the Database
To carry out such a task, the most streamlined solution that can be adopted is to use three custom queries.
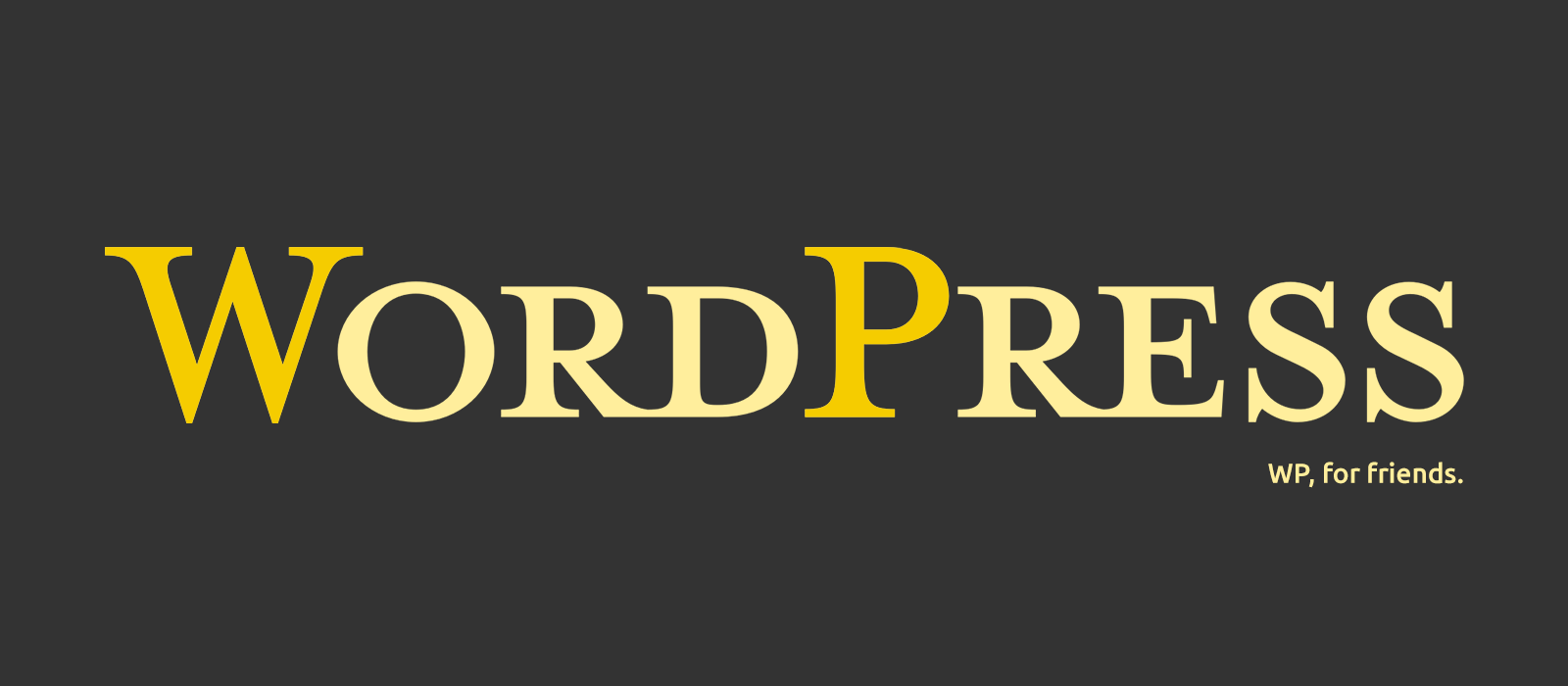
To carry out such a task, there is no utility function made up on purpose in WordPress. After all, deleting all the data associated to a taxonomy isn't a common task, or at least not something you routinely do. Me, for example, I ran into the problem while writing the uninstall.php
script for SiteTree Pro 4.0 — a file loaded when not all the WordPress functions are available, either. But even considering a more generic context, the most streamlined solution that can be adopted is to use three custom queries:
1global $wpdb;
2
3$taxonomy_name = 'the_taxonomy_to_delete';
4
5# Deletes all the terms associated to the taxonomy.
6#
7# The inner query collects from the 'term_taxonomy'
8# table all the ids of the terms to delete.
9$wpdb->query(
10 "DELETE FROM {$wpdb->terms} AS t
11 WHERE t.term_id IN (
12 SELECT tt.term_id FROM {$wpdb->term_taxonomy} AS tt
13 WHERE tt.taxonomy = '{$taxonomy_name}'
14 )"
15);
16
17# Deletes the information used by WordPress
18# to link each post to their terms.
19#
20# Here too, a nested query is needed,
21# and the 'term_taxonomy' table is yet again
22# the source of the data passed to the outer query.
23# But in this case to be collected by
24# the inner query are the ids of
25# the Relationships to delete.
26$wpdb->query(
27 "DELETE FROM {$wpdb->term_relationships} AS tr
28 WHERE tr.term_taxonomy_id IN (
29 SELECT tt.term_taxonomy_id FROM {$wpdb->term_taxonomy} AS tt
30 WHERE tt.taxonomy = '{$taxonomy_name}'
31 )"
32);
33
34# Deletes the base information about the taxonomy.
35$wpdb->query(
36 "DELETE FROM {$wpdb->term_taxonomy}
37 WHERE taxonomy = '{$taxonomy_name}'"
38);
The database must be queried in three distinct times not only because three are the tables from where to delete data, but mainly because two of them, terms
and term_relationships
, depend on the third, term_taxonomy
.